Thank you for your response, I appreciate it. I did as you said, and I am still not getting the values. I took the liberty of just converting the object into a JSON response so I can see it in the Terminal window of Visual Code.
Here is my form:
Here is the actual request header information:
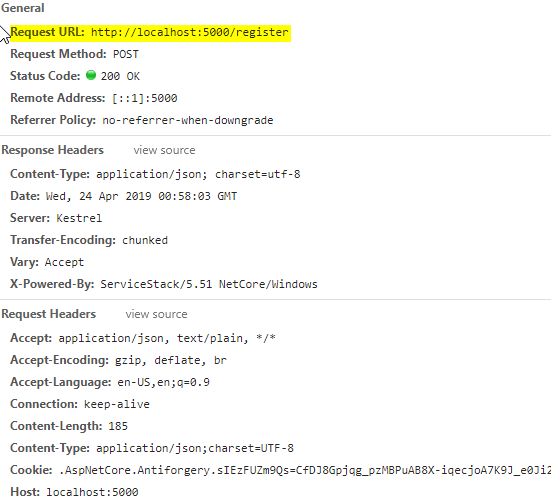
Here is the payload:
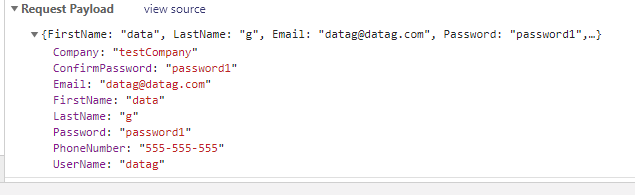
And here is what is coming through to the OnRegistered event:
Request starting HTTP/1.1 POST http://localhost:5000/register application/json;charset=UTF-8 185
Dto Value
{“UserName”:“datag”,“FirstName”:“data”,“LastName”:“g”,“DisplayName”:null,“Email”:“datag@datag.com”,“Password”:“password1”,“ConfirmPassword”:“password1”,“AutoLogin”:null,“Continue”:null,“ErrorView”:null}
Form Data
[]
When I use this code:
public override void OnRegistered(IRequest httpReq, IAuthSession session, IServiceBase registrationService)
{
base.OnRegistered(httpReq, session, registrationService);
var dtoValue = httpReq.Dto as Register;
Console.WriteLine(“Dto Value”);
Console.WriteLine(Helpers.Helper.Dump(dtoValue));
Console.WriteLine(“Form Data”);
Console.WriteLine(Helpers.Helper.Dump(httpReq.FormData));
}
Please advise.
EDIT
Here is what the session object is returning:
{“ReferrerUrl”:null,“Id”:“5o0znLNf45eXrMnIUt5Z”,“UserAuthId”:“1”,“UserAuthName”:null,“UserName”:“datag”,“TwitterUserId”:null,“TwitterScreenName”:null,“FacebookUserId”:null,“FacebookUserName”:null,“FirstName”:“data”,“LastName”:“g”,“DisplayName”:null,“Company”:null,“Email”:“datag@datag.com”,“PrimaryEmail”:“datag@datag.com”,“PhoneNumber”:null,“BirthDate”:null,“BirthDateRaw”:null,“Address”:null,“Address2”:null,“City”:null,“State”:null,“Country”:null,“Culture”:null,“FullName”:null,“Gender”:null,“Language”:null,“MailAddress”:null,“Nickname”:null,“PostalCode”:null,“TimeZone”:null,“RequestTokenSecret”:null,“CreatedAt”:“2019-04-24T01:26:43.3339518Z”,“LastModified”:“2019-04-24T01:26:43.3339518Z”,“Roles”:[],“Permissions”:[],“IsAuthenticated”:false,“FromToken”:false,“ProfileUrl”:null,“Sequence”:null,“Tag”:0,“AuthProvider”:null,“ProviderOAuthAccess”:[],“Meta”:null,“Audiences”:null,“Scopes”:null,“Dns”:null,“Rsa”:null,“Sid”:null,“Hash”:null,“HomePhone”:null,“MobilePhone”:null,“Webpage”:null,“EmailConfirmed”:null,“PhoneNumberConfirmed”:null,“TwoFactorEnabled”:null,“SecurityStamp”:null,“Type”:null}