OK that makes sense. I an easy way to make that manageable.
If I use UserManager like:
await userManager.AddClaimAsync(adminUser, new Claim(JwtClaimTypes.Permission, "CanViewReport"));
It persists it to the AspNetUserClaims table but I don’t see any way to manage that with SS.
I came up with extending the Application User like:
public class ApplicationUser : IdentityUser
{
// ..
public List<string> Permissions { get; set; } = new List<string>();
}
Then inside AdditionalUserClaimsPrincipalFactory
I populate the claims like:
public override async Task<ClaimsPrincipal> CreateAsync(ApplicationUser user)
{
var principal = await base.CreateAsync(user);
var identity = (ClaimsIdentity)principal.Identity!;
var claims = new List<Claim>();
if (user.Permissions?.Count > 0)
{
foreach(var permission in user.Permissions)
{
claims.Add(new Claim(JwtClaimTypes.Permissions, permission));
}
}
identity.AddClaims(claims);
return principal;
}
And then I can add this to the /admin-ui
edit form like so:
options.AdminUsersFeature(feature =>
{
feature.FormLayout =
[
// ..
Input.For<ApplicationUser>(x => x.Permissions, c => c.Type = Input.Types.Tag),
];
});
Does this seem like an OK solution? I am always a bit trepidations when it comes to auth so appreciate any input.
Also, I noticed in the components gallery there is a multi-select:
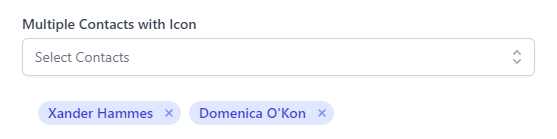
Is it possible to use that in locode forms? It would be a much nicer solution as the free-form tag inputs leave some room for error.
I tried with Combobox
but can’t see a multiselect option:
Input.For<ApplicationUser>(x => x.Permissions, c => {
c.Type = Input.Types.Combobox;
c.AllowableEntries = new KeyValuePair<string, string>[]
{
new KeyValuePair<string, string>("Can View Reports", "CanViewReport"),
new KeyValuePair<string, string>("Can Delete Reports", "CanDeleteReport"),
};
}),
Many thanks for all the support and advice!